Data Tables
DataTables is a plug-in for the jQuery Javascript library. It is a highly flexible tool, based upon the foundations of progressive enhancement, and will add advanced interaction controls to any HTML table.
Page Demo Plugin WebsiteDataTable features:
- Pagination, instant search and multi-column ordering.
- Supports almost any data source: DOM, Javascript, Ajax and server-side processing
- Wide variety of extensions inc. Editor, Buttons, FixedColumns and more
- Extensive options and a beautiful, expressive API
- Fully internationalisable
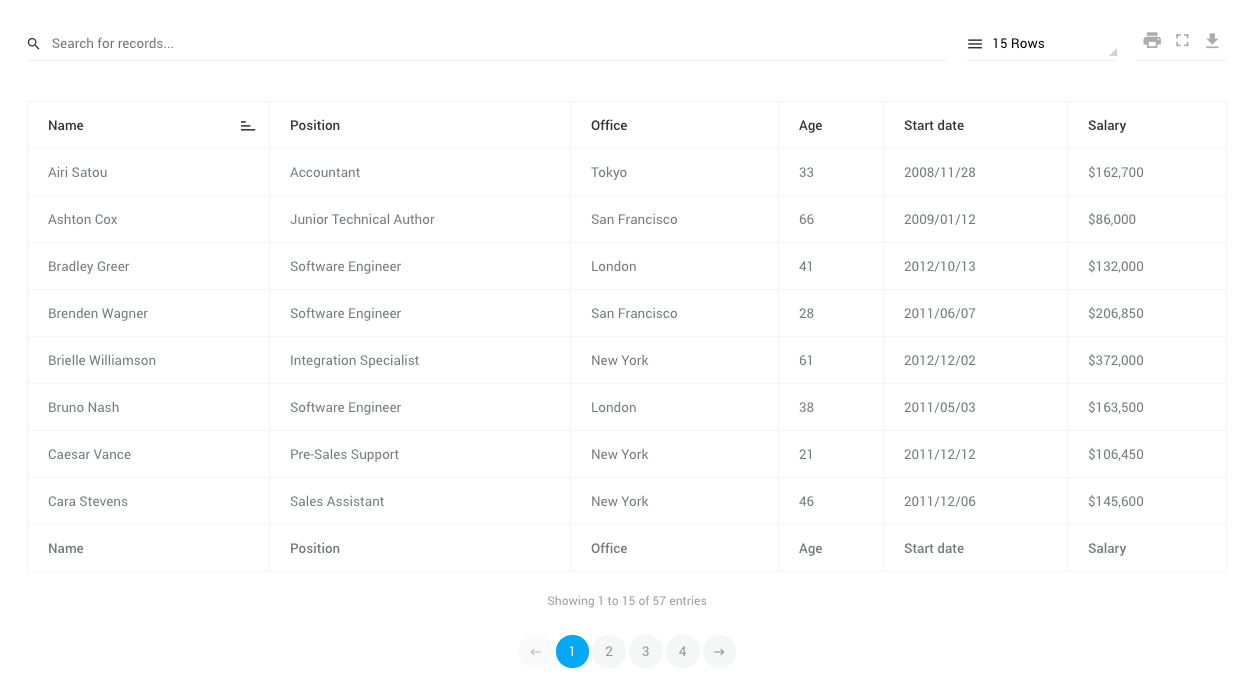
<table id="data-table" class="table">
<thead>
<tr>
<th>Name</th>
<th>Position</th>
<th>Office</th>
<th>Age</th>
<th>Start date</th>
<th>Salary</th>
</tr>
</thead>
<tbody>
<tr>
<td>Tiger Nixon</td>
<td>System Architect</td>
<td>Edinburgh</td>
<td>61</td>
<td>2011/04/25</td>
<td>$320,800</td>
</tr>
<tr>
<td>Garrett Winters</td>
<td>Accountant</td>
<td>Tokyo</td>
<td>63</td>
<td>2011/07/25</td>
<td>$170,750</td>
</tr>
...
</tbody>
</table>
// Please note that this function is already included in js/app.min.js(from js/inc/functions/vendors.js)
// thus you don't need to add this again.
if($('#data-table')[0]) {
// Add custom buttons
var dataTableButtons = '<div class="dataTables_buttons hidden-sm-down actions">' +
'<span class="actions__item zmdi zmdi-print" data-table-action="print" />' +
'<span class="actions__item zmdi zmdi-fullscreen" data-table-action="fullscreen" />' +
'<div class="dropdown actions__item">' +
'<i data-toggle="dropdown" class="zmdi zmdi-download" />' +
'<ul class="dropdown-menu dropdown-menu-right">' +
'<a href="" class="dropdown-item" data-table-action="excel">Excel (.xlsx)</a>' +
'<a href="" class="dropdown-item" data-table-action="csv">CSV (.csv)</a>' +
'</ul>' +
'</div>' +
'</div>';
// Initiate data-table
$('#data-table').DataTable({
autoWidth: false,
responsive: true,
lengthMenu: [[15, 30, 45, -1], ['15 Rows', '30 Rows', '45 Rows', 'Everything']], //Length select
language: {
searchPlaceholder: "Search for records..." // Search placeholder
},
dom: 'Blfrtip',
buttons: [ // Data table buttons for export and print
{
extend: 'excelHtml5',
title: 'Export Data'
},
{
extend: 'csvHtml5',
title: 'Export Data'
},
{
extend: 'print',
title: 'Material Admin'
}
],
"initComplete": function(settings, json) {
$(this).closest('.dataTables_wrapper').prepend(dataTableButtons); // Add custom button (fullscreen, print and export)
}
});
// Data table button actions
$('body').on('click', '[data-table-action]', function (e) {
e.preventDefault();
var exportFormat = $(this).data('table-action');
if(exportFormat === 'excel') {
$(this).closest('.dataTables_wrapper').find('.buttons-excel').trigger('click');
}
if(exportFormat === 'csv') {
$(this).closest('.dataTables_wrapper').find('.buttons-csv').trigger('click');
}
if(exportFormat === 'print') {
$(this).closest('.dataTables_wrapper').find('.buttons-print').trigger('click');
}
if(exportFormat === 'fullscreen') {
var parentCard = $(this).closest('.card');
if(parentCard.hasClass('card--fullscreen')) {
parentCard.removeClass('card--fullscreen');
$('body').removeClass('data-table-toggled');
}
else {
parentCard.addClass('card--fullscreen')
$('body').addClass('data-table-toggled');
}
}
});
}
Requirements:
/scss/inc/vendor-overrides/_data-tables.scss
/js/inc/functions/vendors.js
/vendors/datatables/js/jquery.dataTables.min.js
/vendors/datatables-buttons/js/dataTables.buttons.min.js
/vendors/datatables-buttons/js/buttons.print.min.js
/vendors/jszip/dist/jszip.min.js
/vendors/datatables-buttons/js/buttons.html5.min.js